목차
playwright 란?
javascript, python 으로 웹 페이지를 테스팅할 수 있게 해주는 라이브러리
( 본 게시글에서는 Node.js를 사용합니다. )
playwright 설치
// 기본 패키지 설치
npm i playwright
// 테스트를 위한 패키지 설치
npm i @playwright/test
playwright 프로젝트 만들기 ( Node.js )
npm init playwright
테스트 파일 만들기
// playwright/tests/example.spec.ts
import { test, expect } from "@playwright/test";
test("basic test", async ({ page }) => {
// playwright 공식페이지로 이동한다. - goto(url)
await page.goto("https://playwright.dev/");
// 페이지에서 클래스를 찾고, 변수에 저장한다. - locator("태그 or 클래스 or ID")
const title = page.locator(".navbar__inner .navbar__title");
// 저장된 부분에 "Playwright"문자열이 들어있는지 테스트 하고 결과 보여주기 - expect().toHaveText()
await expect(title).toHaveText("Playwright");
});
테스트 파일 실행하기
// headless 방식으로 테스트하기
npx playwright test
// 브라우저 사용해서 테스트하기 ( 기본 : chromium )
npx playwright test --headed
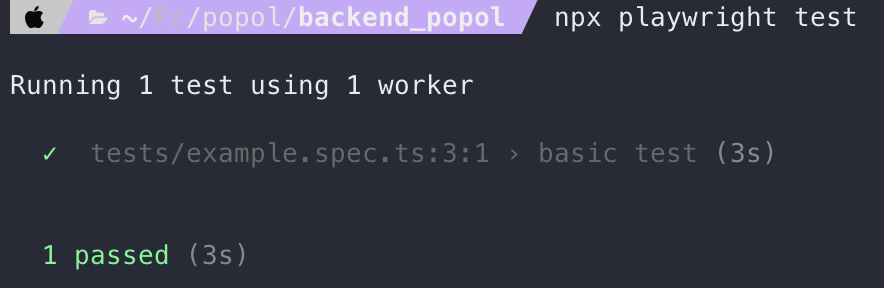
playwright 설정 파일 만들기
// playwright/playwright.config.ts - 글로벌(전역)으로 적용된다!
// typescript에서 사용하기 위한 타입, 테스팅 브라우저 설정할 모듈 불러오기
import { PlaywrightTestConfig, devices } from "@playwright/test";
const config: PlaywrightTestConfig = {
forbidOnly: !!process.env.CI,
retries: process.env.CI ? 2 : 0, //실패시 재시도 횟수 설정
use: {
trace: "on-first-retry",
},
projects: [
{
name: "chromium",
use: { ...devices["Desktop Chrome"] },
}, // Desktop Chrome 브라우저 사용해서 테스트
{
name: "firefox",
use: { ...devices["Desktop Firefox"] },
},
{
name: "webkit",
use: { ...devices["Desktop Safari"] },
},
],
};
export default config;
반응형
playwright 란?
javascript, python 으로 웹 페이지를 테스팅할 수 있게 해주는 라이브러리
( 본 게시글에서는 Node.js를 사용합니다. )
playwright 설치
// 기본 패키지 설치
npm i playwright
// 테스트를 위한 패키지 설치
npm i @playwright/test
playwright 프로젝트 만들기 ( Node.js )
npm init playwright
테스트 파일 만들기
// playwright/tests/example.spec.ts
import { test, expect } from "@playwright/test";
test("basic test", async ({ page }) => {
// playwright 공식페이지로 이동한다. - goto(url)
await page.goto("https://playwright.dev/");
// 페이지에서 클래스를 찾고, 변수에 저장한다. - locator("태그 or 클래스 or ID")
const title = page.locator(".navbar__inner .navbar__title");
// 저장된 부분에 "Playwright"문자열이 들어있는지 테스트 하고 결과 보여주기 - expect().toHaveText()
await expect(title).toHaveText("Playwright");
});
테스트 파일 실행하기
// headless 방식으로 테스트하기
npx playwright test
// 브라우저 사용해서 테스트하기 ( 기본 : chromium )
npx playwright test --headed
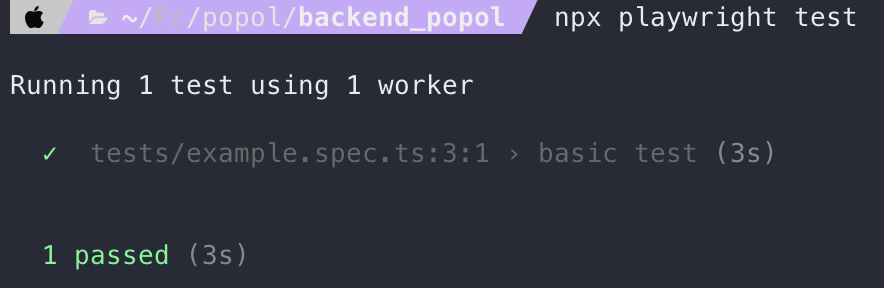
playwright 설정 파일 만들기
// playwright/playwright.config.ts - 글로벌(전역)으로 적용된다!
// typescript에서 사용하기 위한 타입, 테스팅 브라우저 설정할 모듈 불러오기
import { PlaywrightTestConfig, devices } from "@playwright/test";
const config: PlaywrightTestConfig = {
forbidOnly: !!process.env.CI,
retries: process.env.CI ? 2 : 0, //실패시 재시도 횟수 설정
use: {
trace: "on-first-retry",
},
projects: [
{
name: "chromium",
use: { ...devices["Desktop Chrome"] },
}, // Desktop Chrome 브라우저 사용해서 테스트
{
name: "firefox",
use: { ...devices["Desktop Firefox"] },
},
{
name: "webkit",
use: { ...devices["Desktop Safari"] },
},
],
};
export default config;
반응형